JS基础(一)
数据类型
基本类型:
- Boolean;
- Null;
- Undefined;
- Number;
- String;
引用类型 :
6. Objects: Array, function, Date
新增:
7. BigInt: 谷歌67版本出现的数据类型, 是指安全存储、操作大整数,(但是很多人不把这个做为一个类型);
8. Symbol:ES6新增, 这种类型的对象永不相等,即始创建的时候传入相同的值,可以解决属性名冲突的问题,做为标记;
类型判断
- typeof
- instanceof
- Object.prototype.toString
- isXXX,比如 isArray
typeof:返回一个字符串
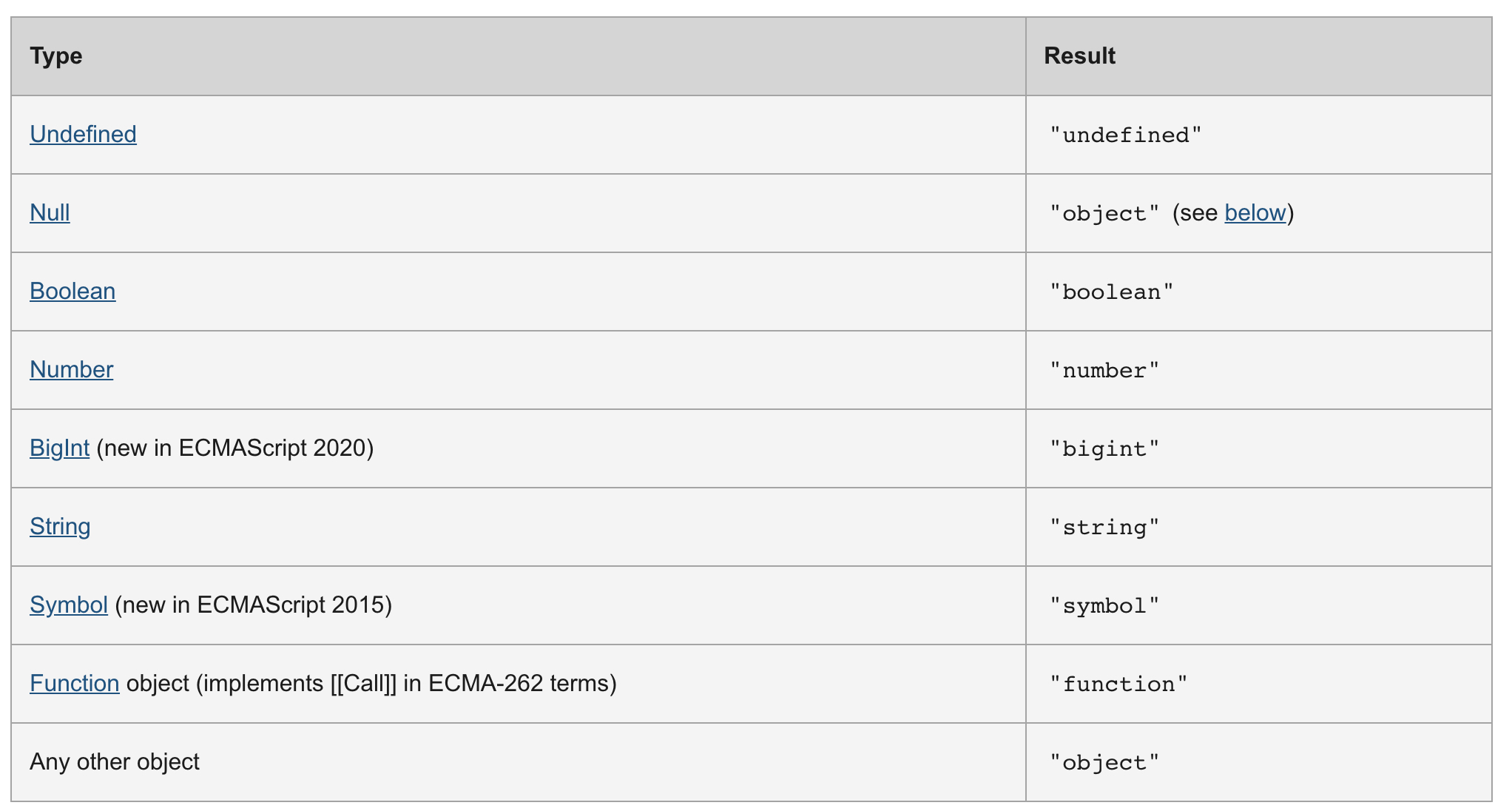
因为Object 下有很多细分的类型,如 Array、Function、Date、RegExp、Error 等,typeof无法一一区分,因此可以用Object.prototype.toString来进一步区分:
When the toString method is called, the following steps are taken:
- If the this value is undefined, return “[object Undefined]”.
- If the this value is null, return “[object Null]”.
- Let O be the result of calling ToObject passing the this value as the argument.
- Let class be the value of the [[Class]] internal property of O.
- Return the String value that is the result of concatenating the three Strings “[object “, class, and “]”.
1 | // 以下是11种: |
instanceof: 用于判断一个变量是否属于某个对象的实例。也可以用来判断某个构造函数的prototype属性是否存在另外一个要检测对象的原型链上。
1 | function test(){};var a=new test();alert(a instanceof test) // true |
参考:
[1]:https://www.php.cn/js-tutorial-411579.html
[2]:https://github.com/mqyqingfeng/Blog/issues/28
-------------本文结束&感谢您的阅读-------------
相关文章